**************************
Voir Readme / TODO
**************************
https://cdn.epic.net/uploads/2022/07/epic-moments_of_happiness-chill_the_lion-loop-ld.mp4



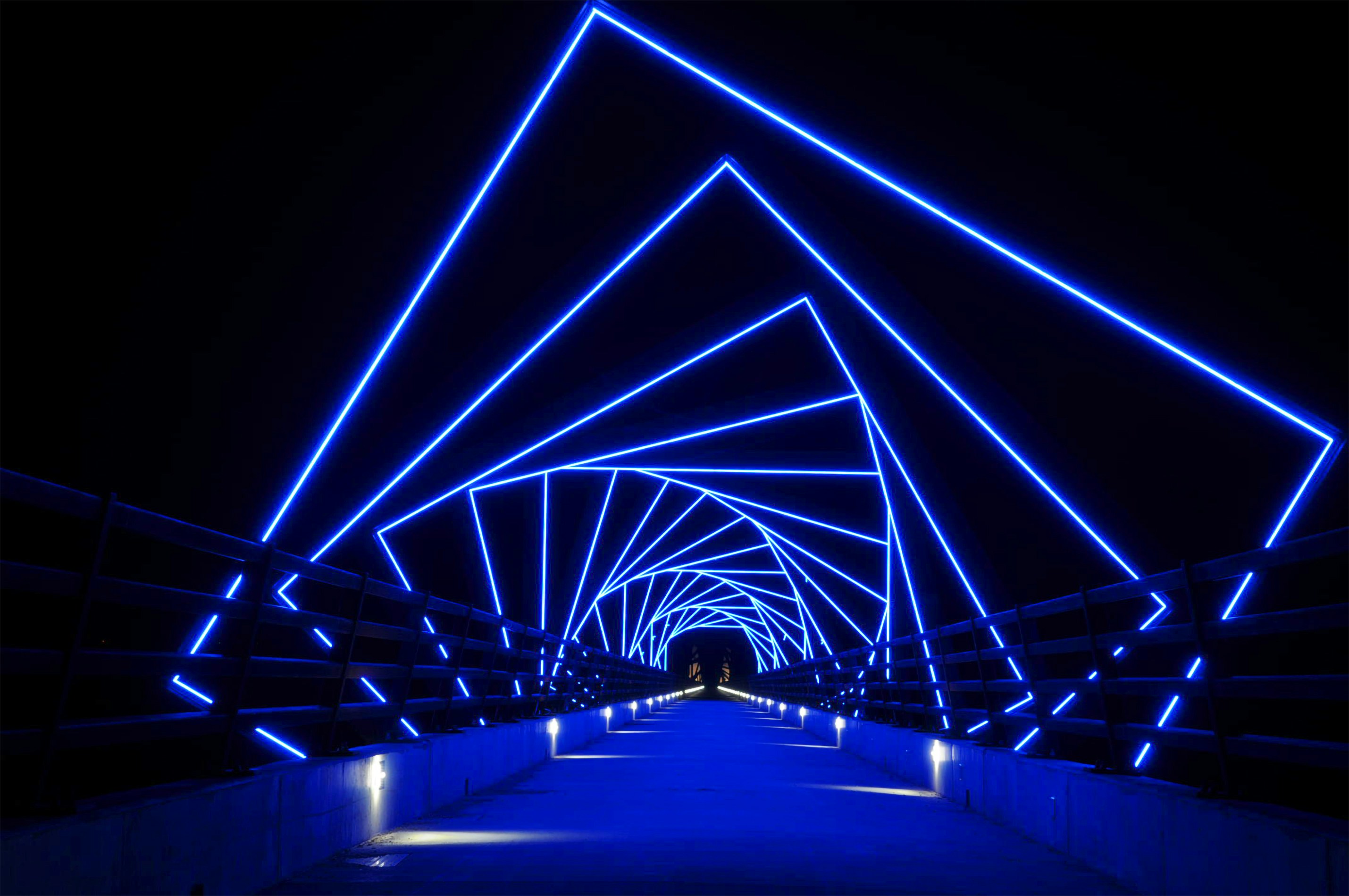
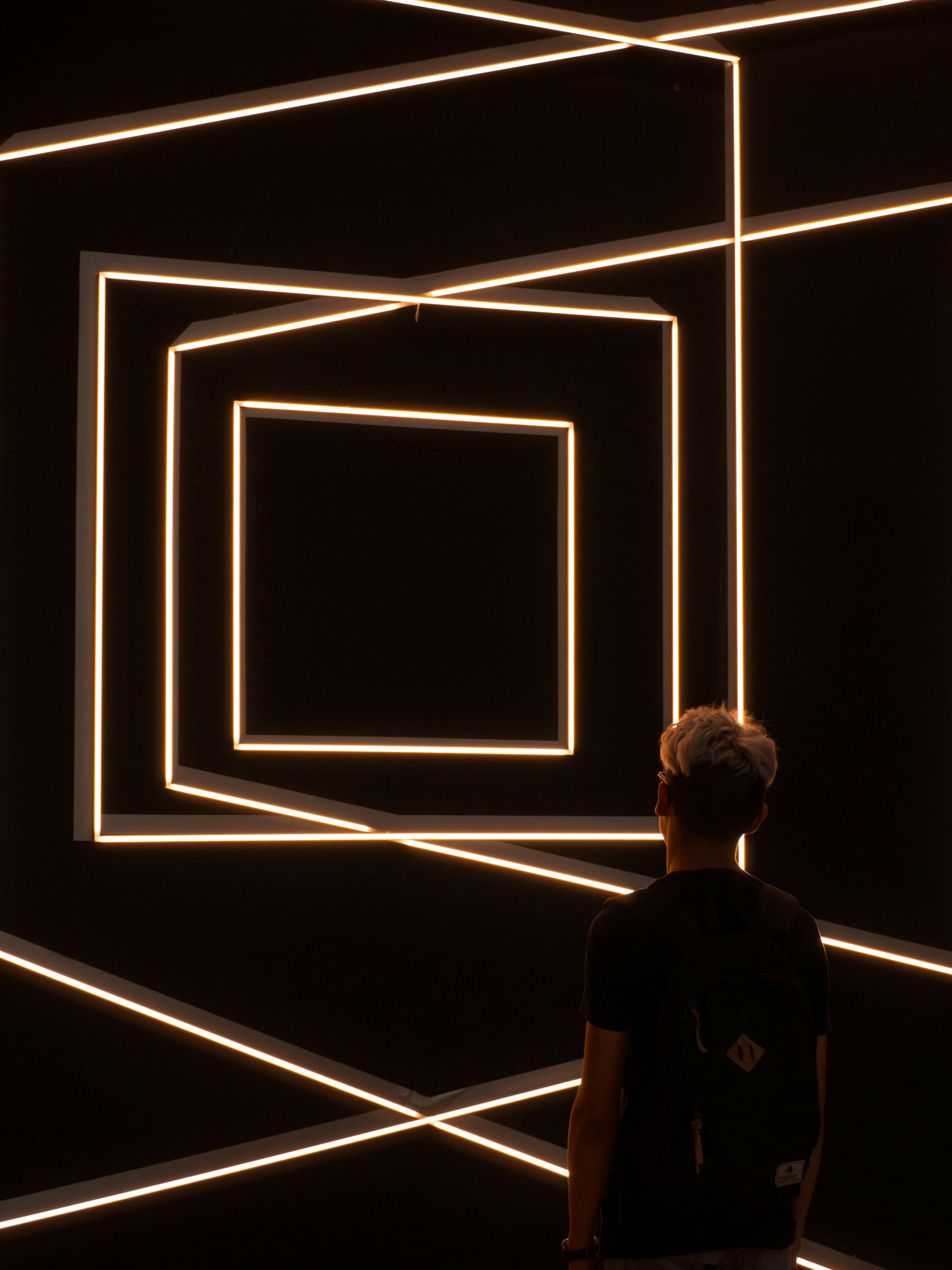
<!-- _footer: cover art "unnamed" by zug https://www.vertexshaderart.com/art/XK2y2Cy63Ez45E7pm
<!-- _footer: image de couverture: https://teia.art/objkt/627718
<!-- _footer: cover art: Mitchell Kavan https://unsplash.com/@mtk
<!-- _footer: photo de couverture: Steven Ramon https://unsplash.com/@ssttevven
https://www.youtube.com/watch?v=zBJXXcFy6iU
--- ## [Utilisation de Parcel](https://parceljs.org/getting-started/webapp/) - appeler le bundler directement ``` npx parcel src/index.html ``` --- - ou modifier `package.json` ```json { "name": "my-project", "source": "src/index.html", "scripts": { "start": "parcel", "build": "parcel build" }, "devDependencies": { "parcel": "latest" } } ``` ``` npm start ```
- https://github.com/torinmb/threejs-parcel-es6-starter - https://github.com/DrSensor/js13k-parcel
https://gitlab.inria.fr/Loki/PolyphonyECS